mongoDB
Robo 3T : MongoDB GUI 工具
download RoBo 3T (for windows .zip version 即可)
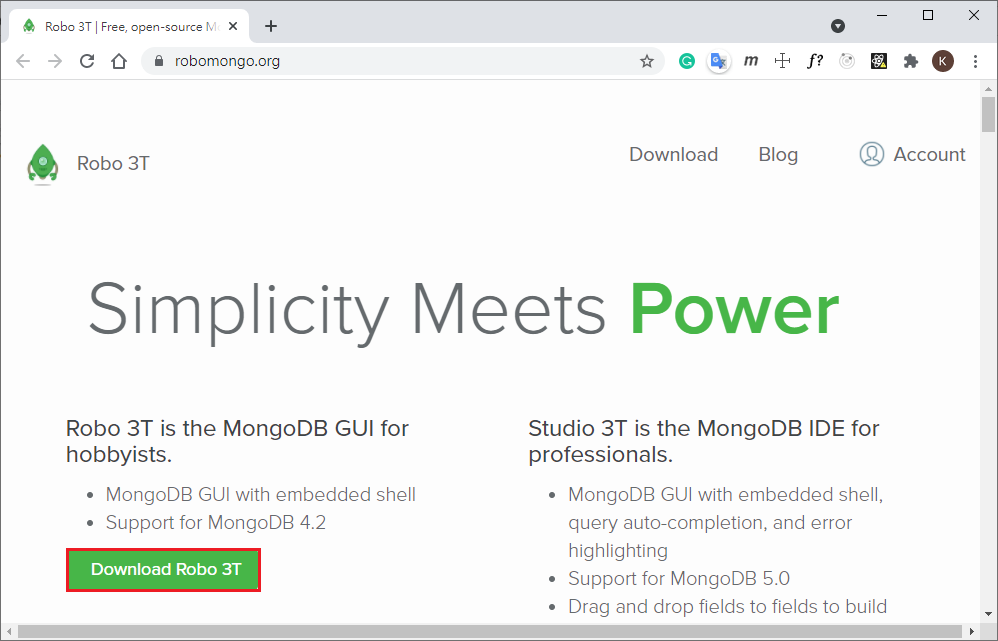
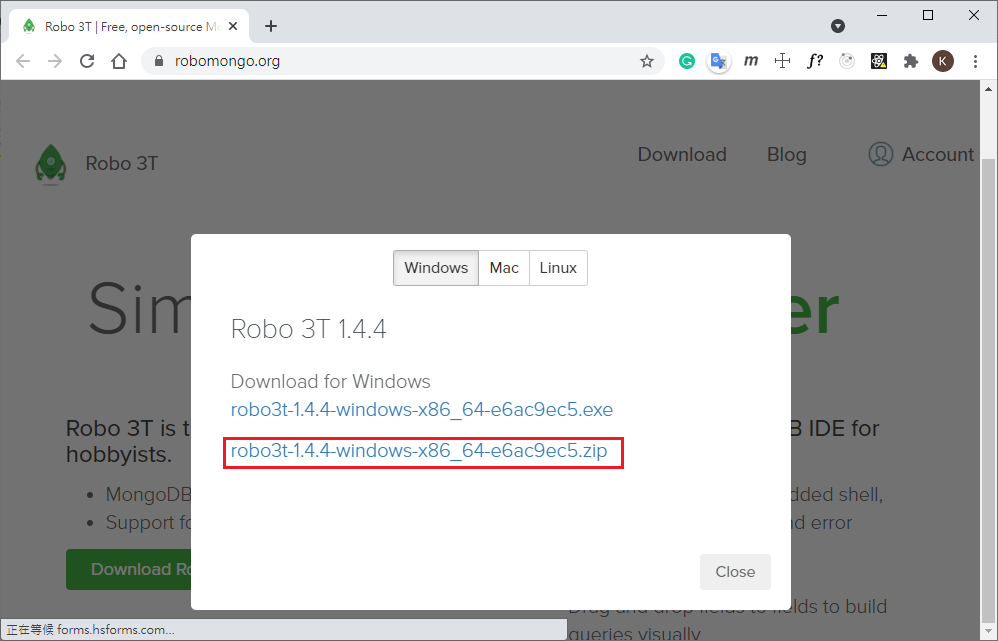
解壓縮後執行 robo3t.exe
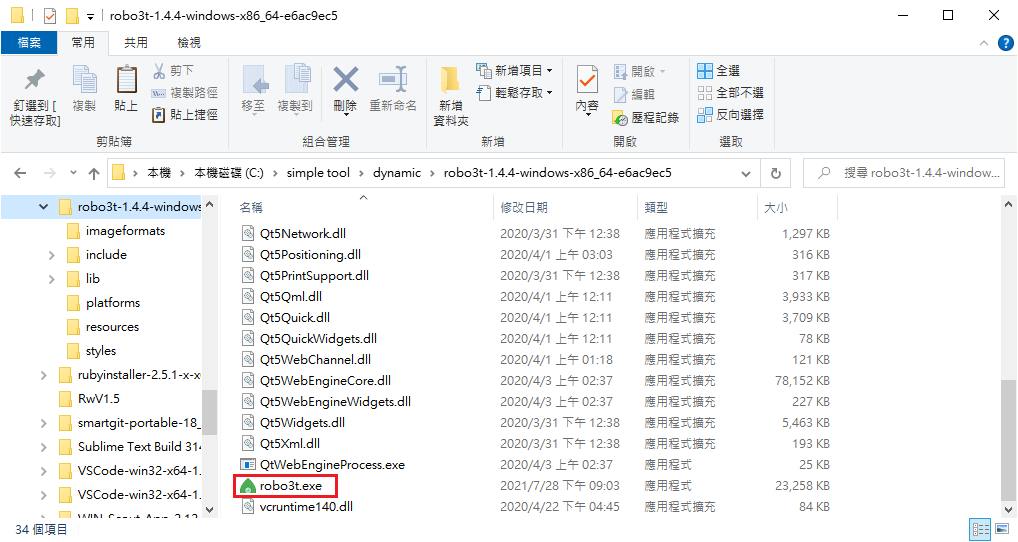
選擇 I agree , Next
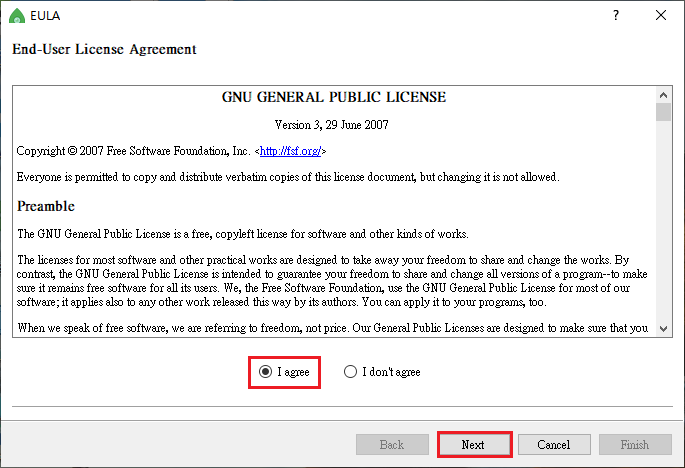
選擇 Finish
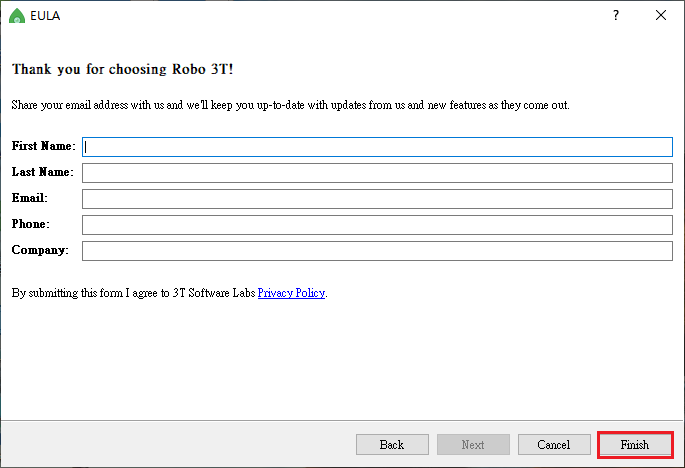
選擇 Create, 填入由MongoDB atlas 取得 url(Node.js 4.0 or later 版本即可), 選 Form URI
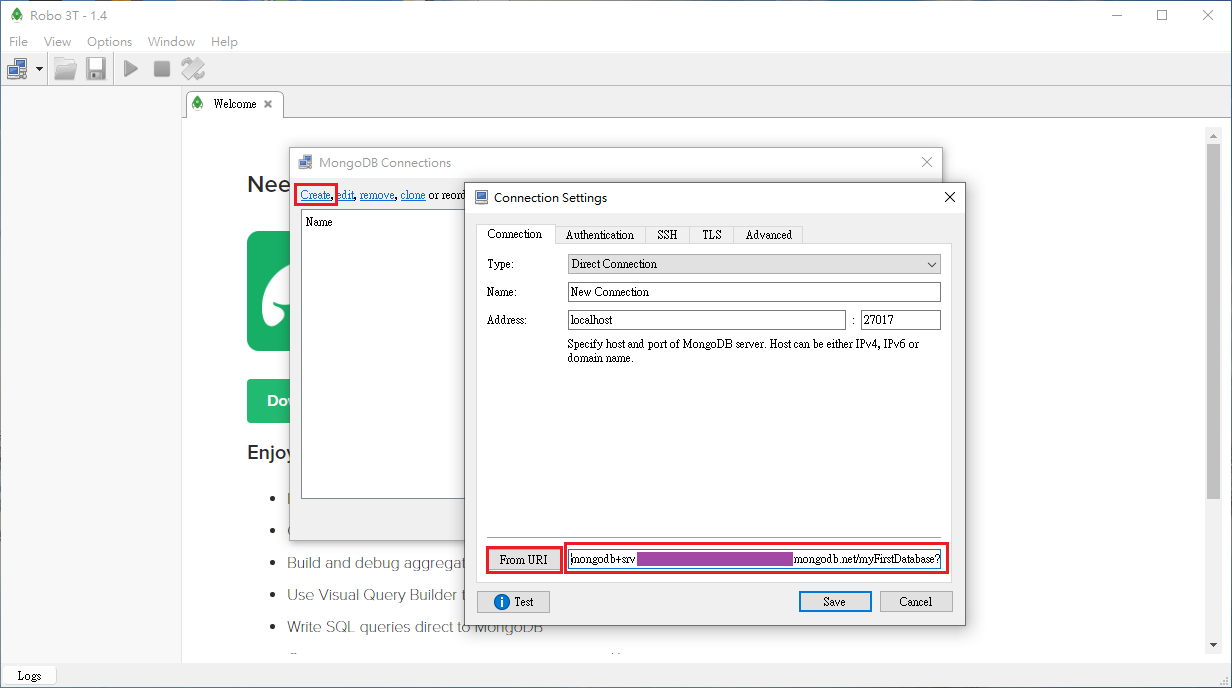
選擇 Save
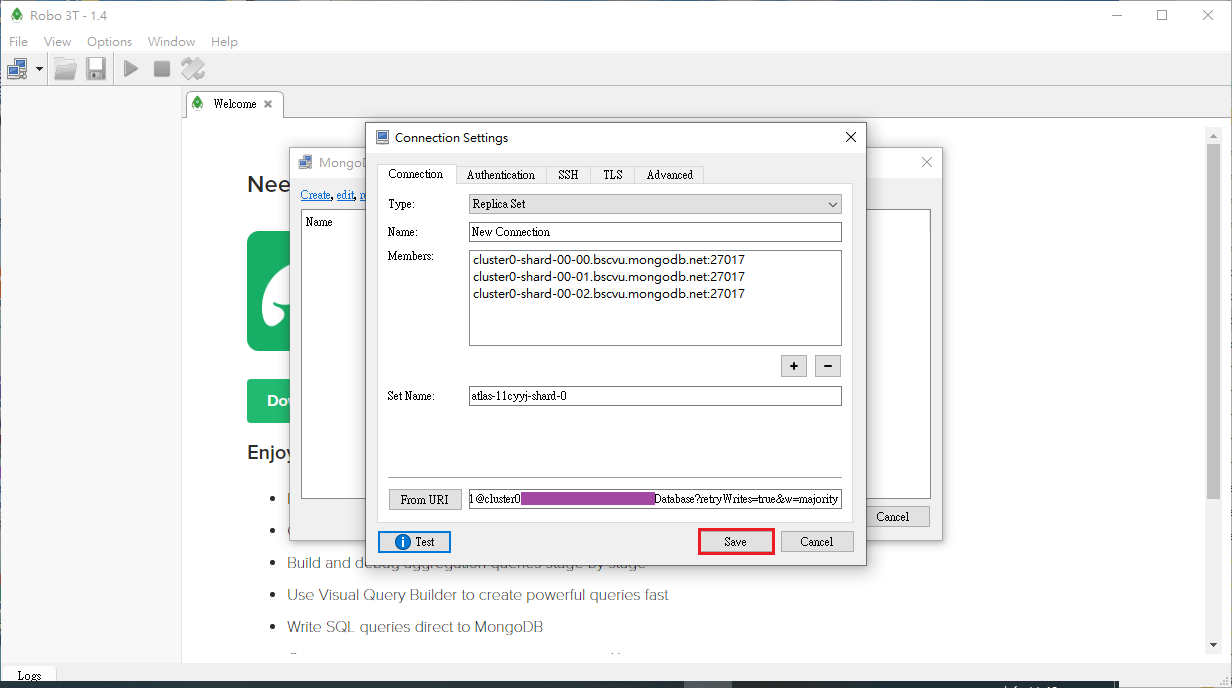
選擇 Connect 即可見到 DB 畫面
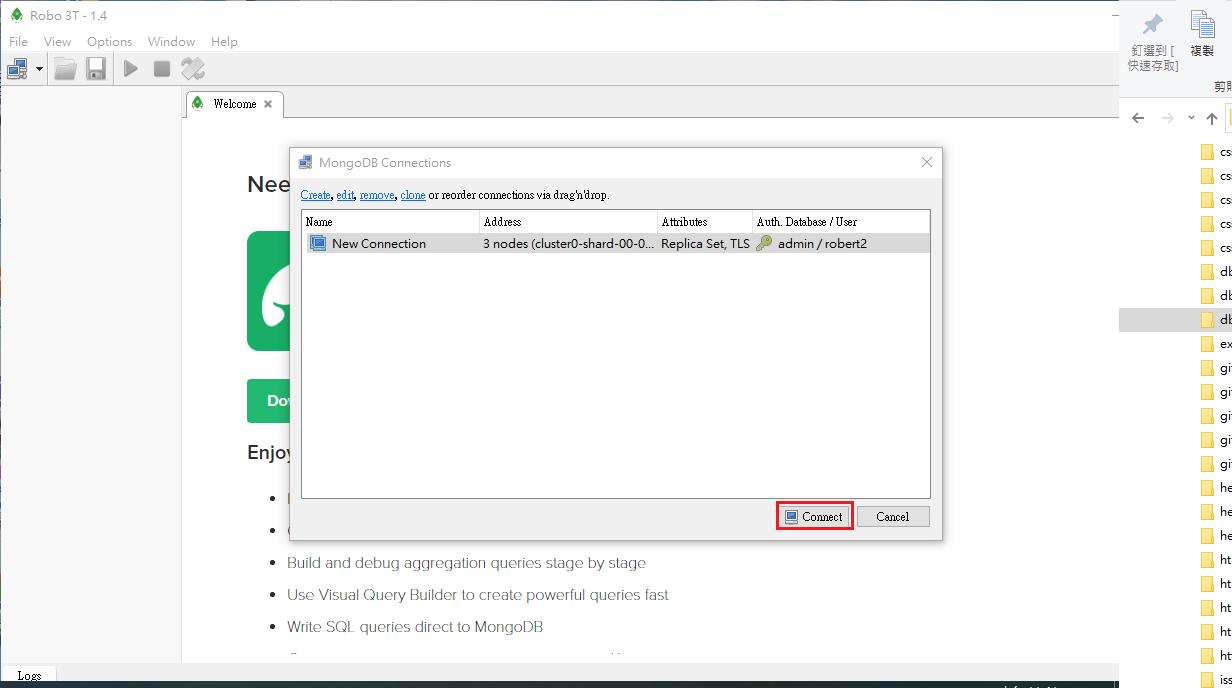
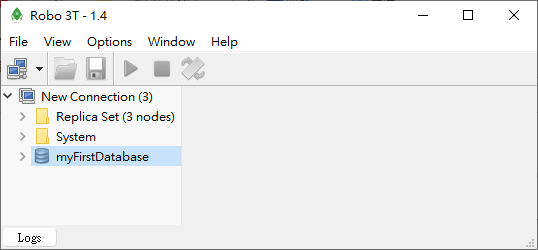
MongoDB Altas add project
選擇 New Project, Next, Create Project
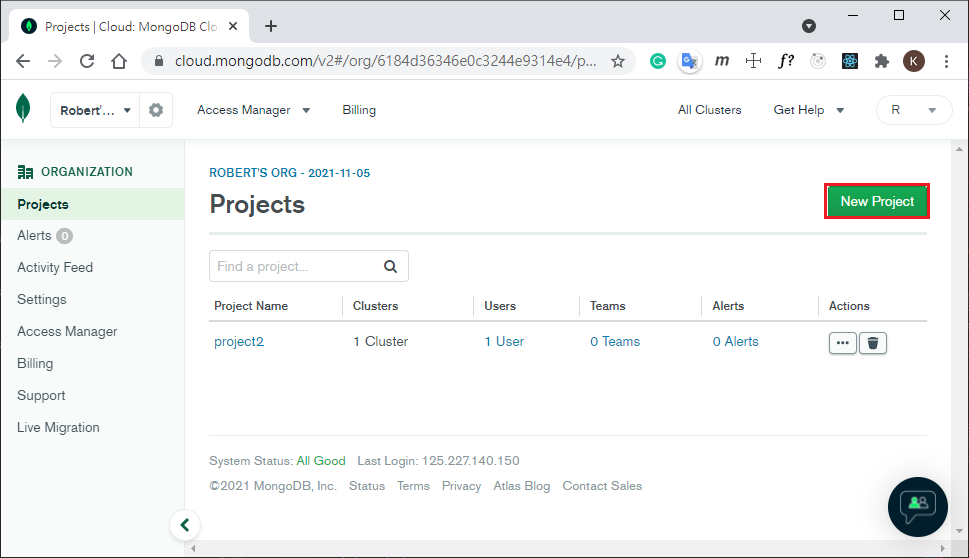
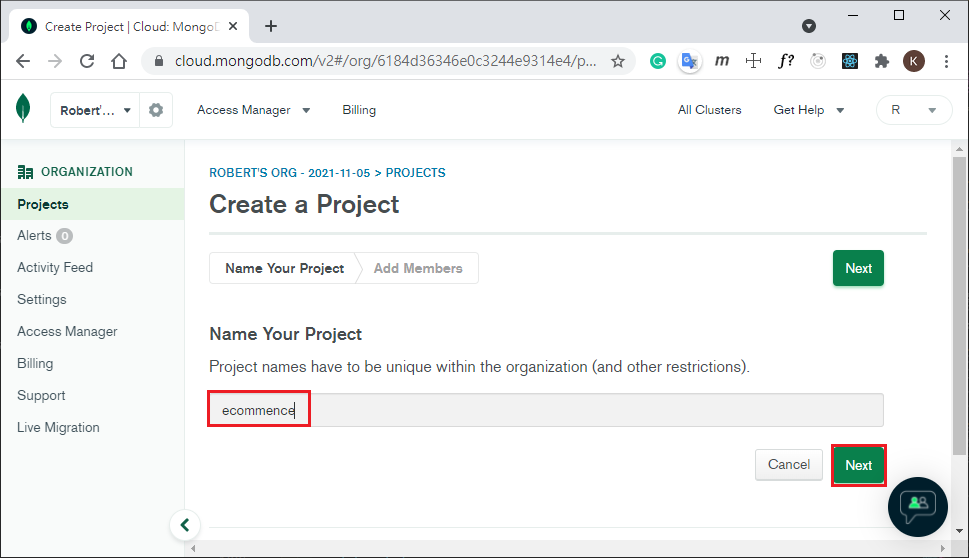
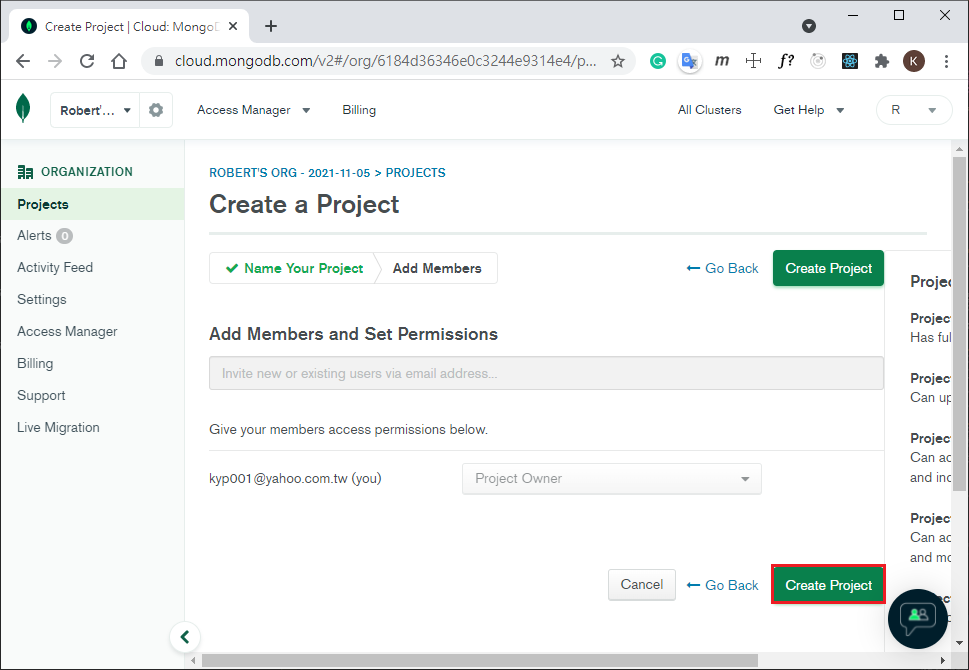
Databases : Build a Database, Shared(Create), select position+Create Cluster
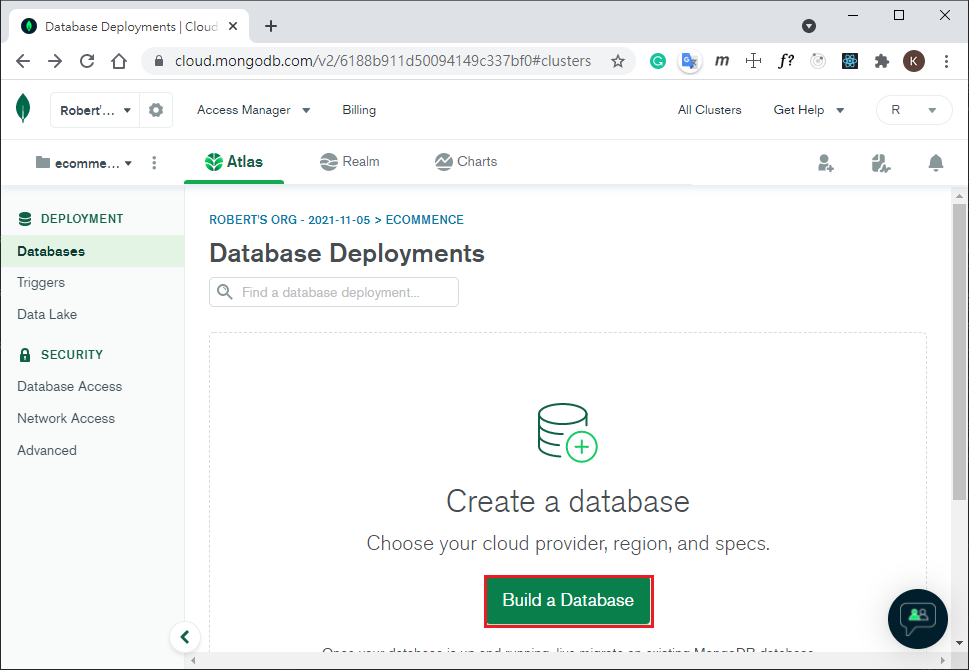
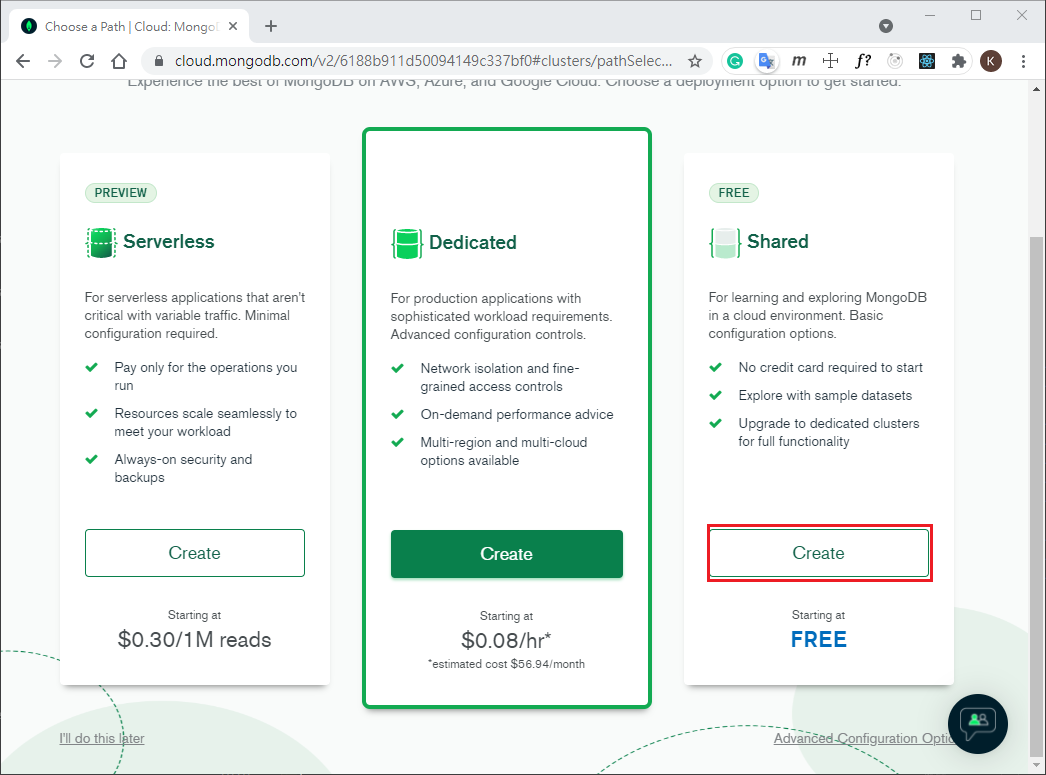
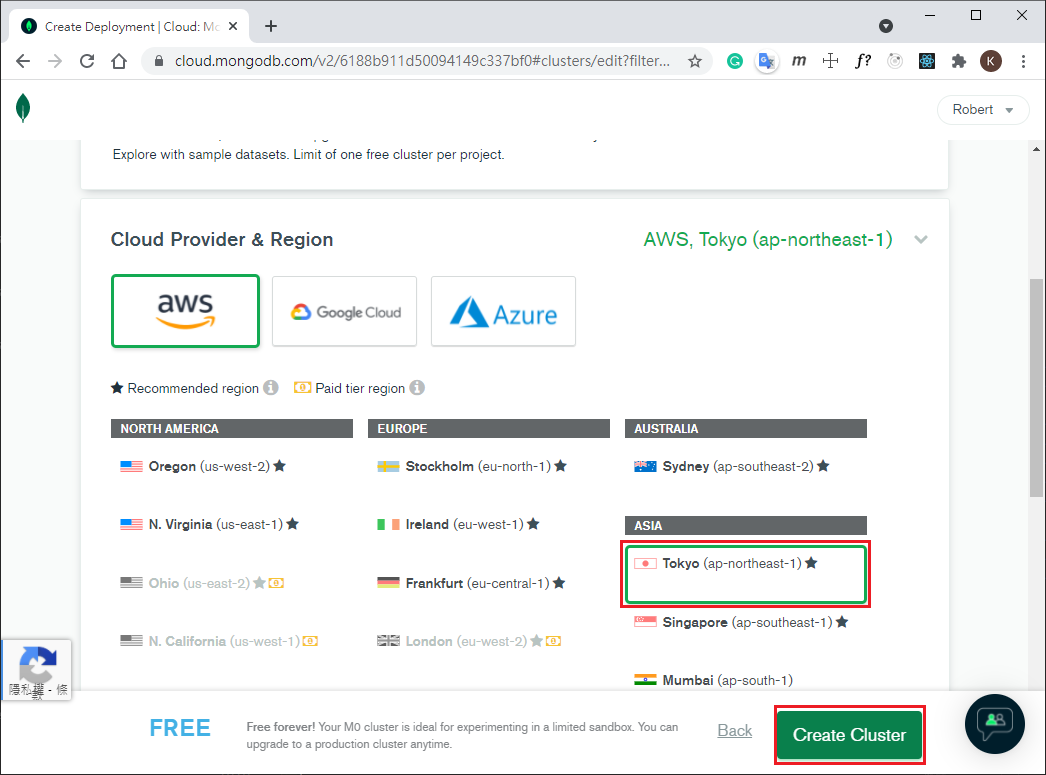
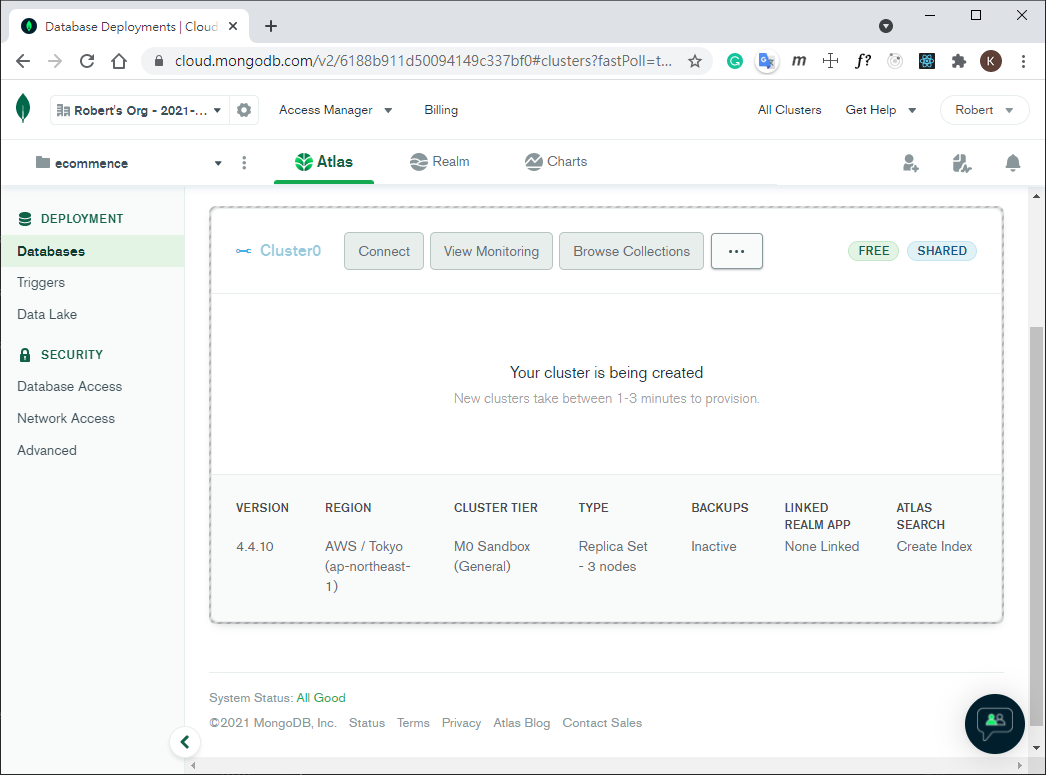
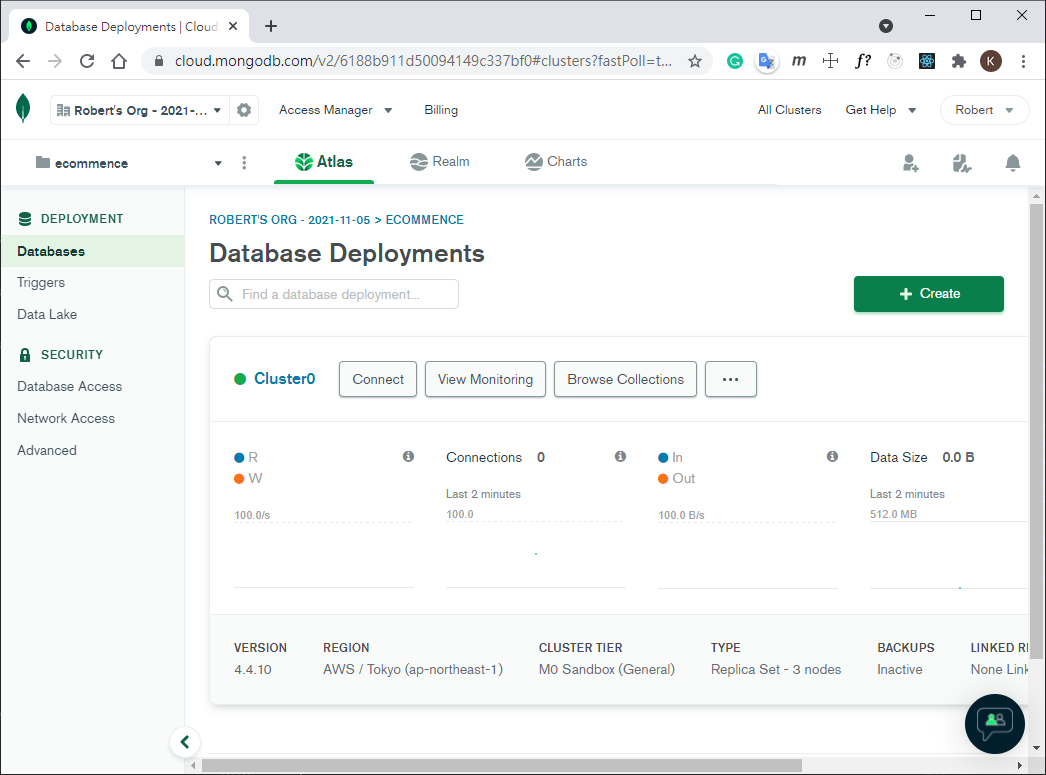
Network Access : Add IP Address, 0.0.0.0/0(表不限制), Confirm
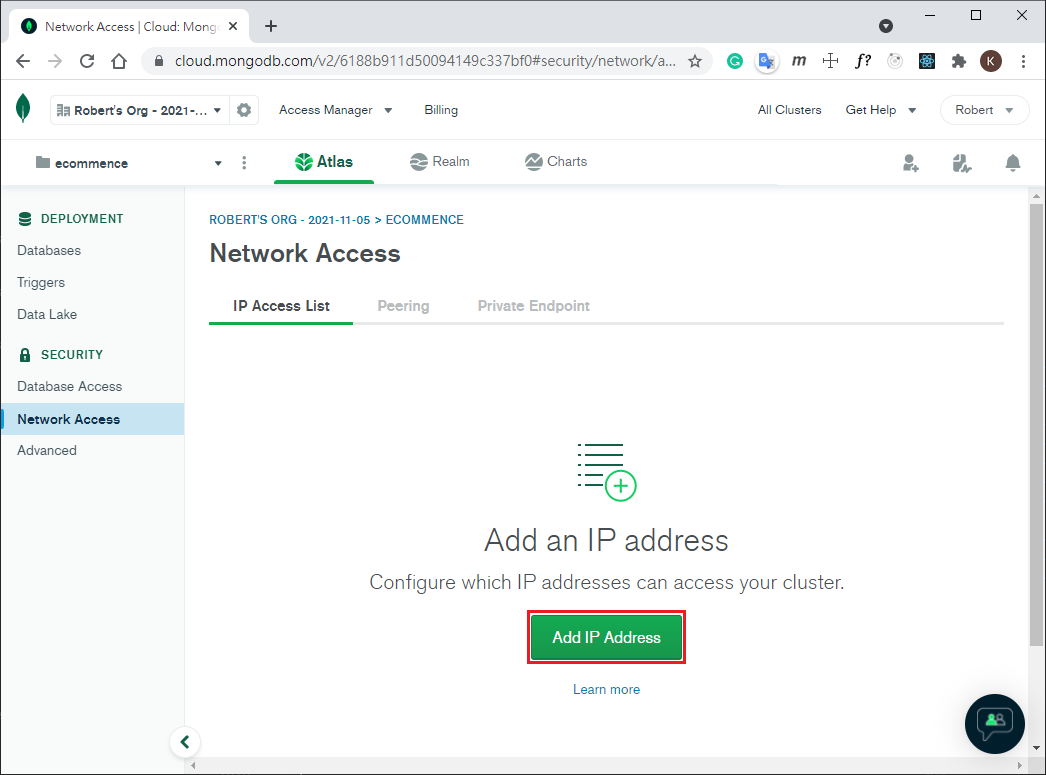
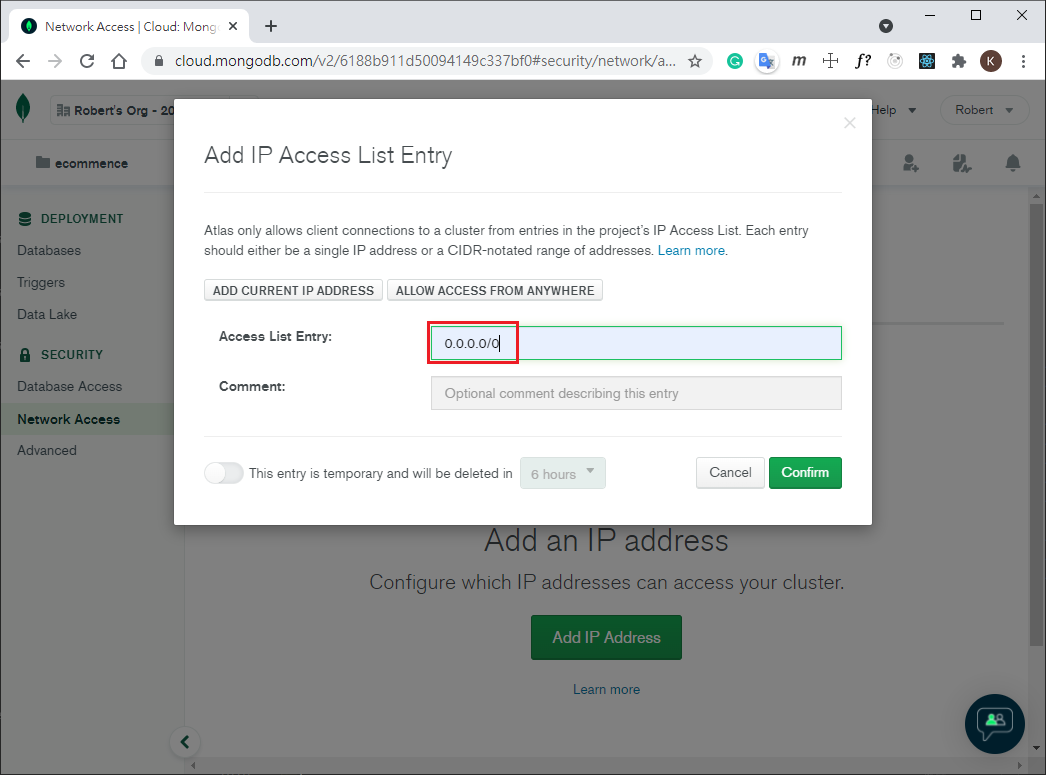
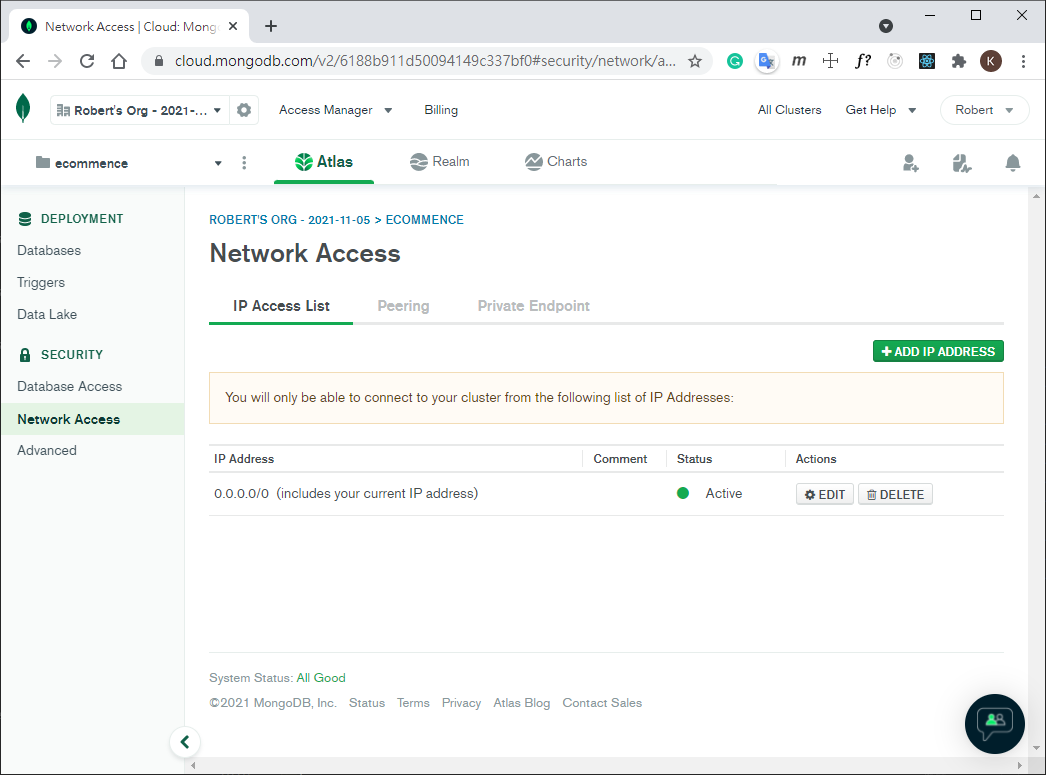
Database Access: add New DataBase User, set user info, add User
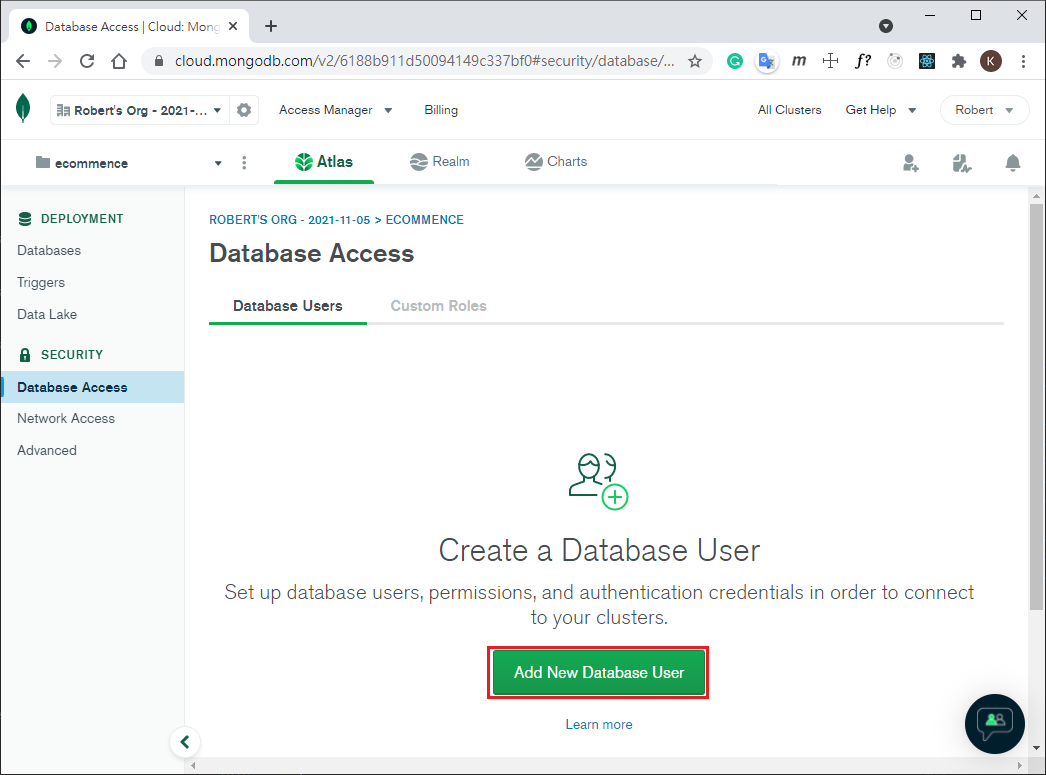
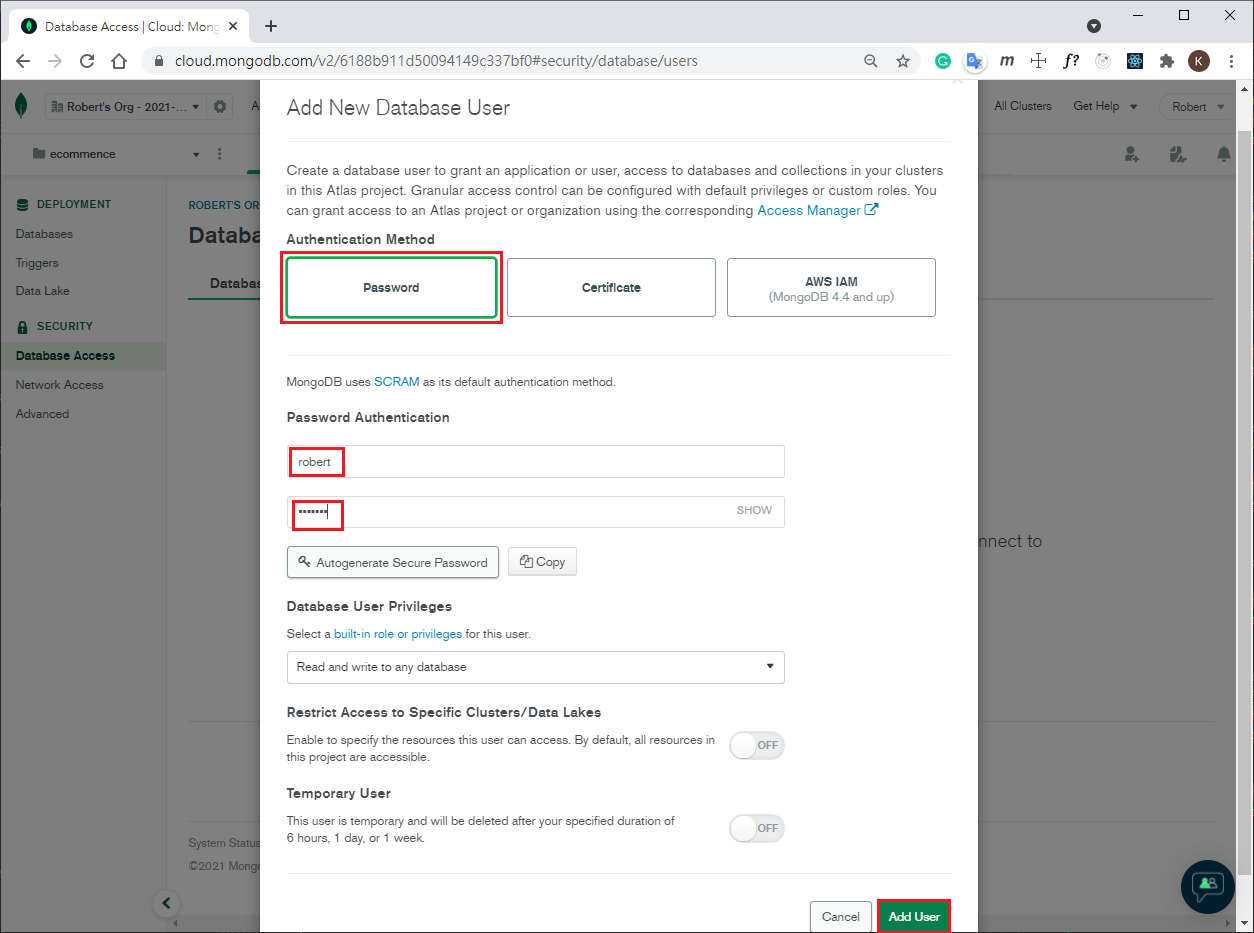
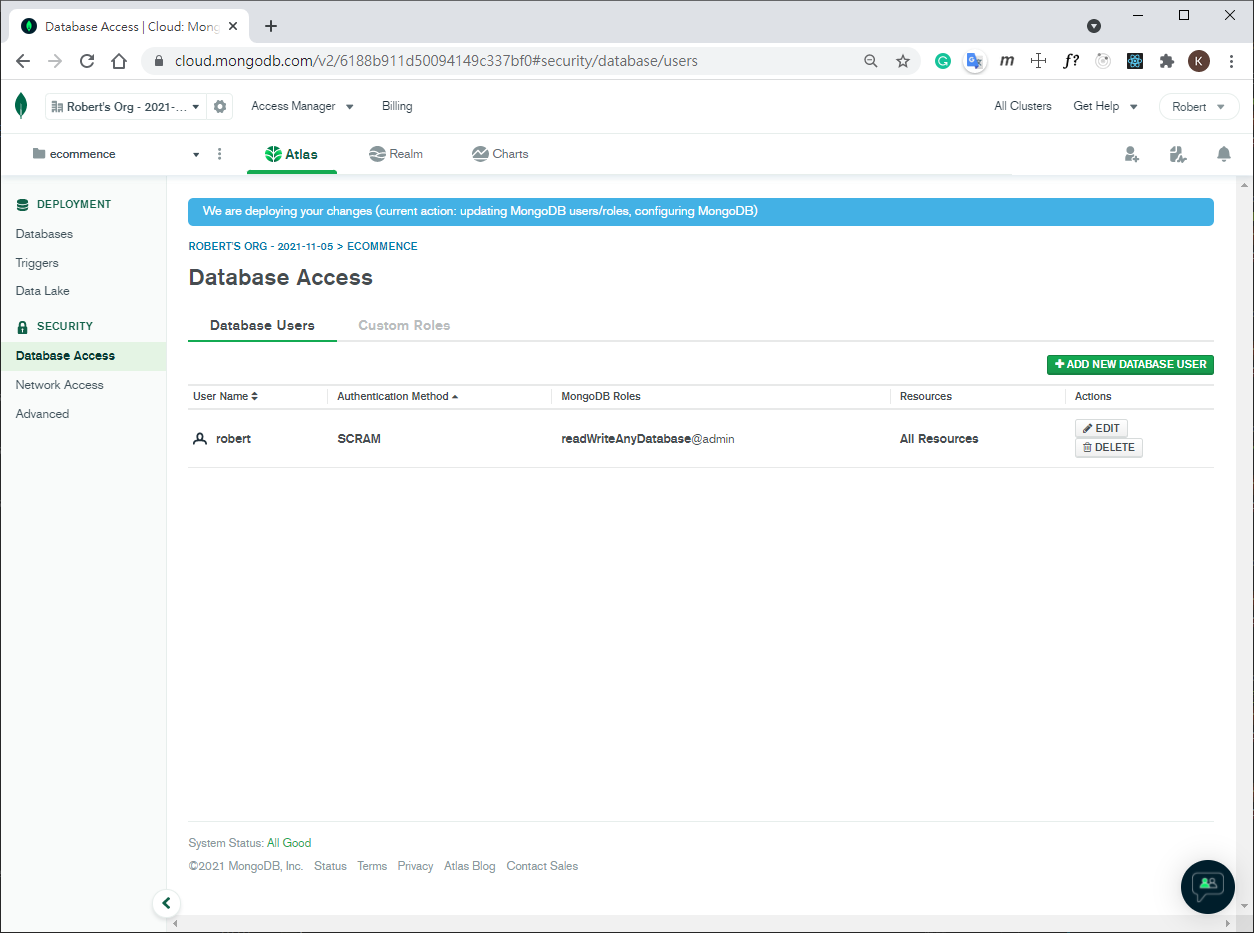
get link url, Databases: Connect, Connect your application,
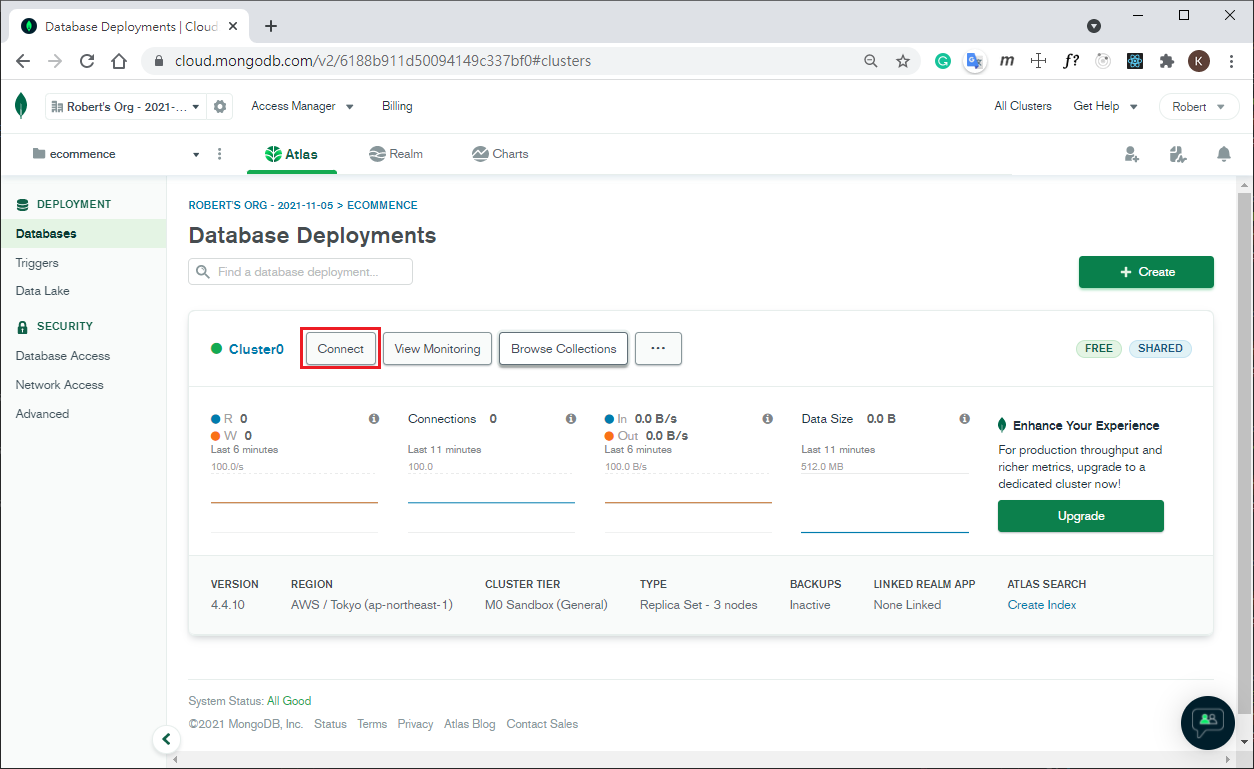
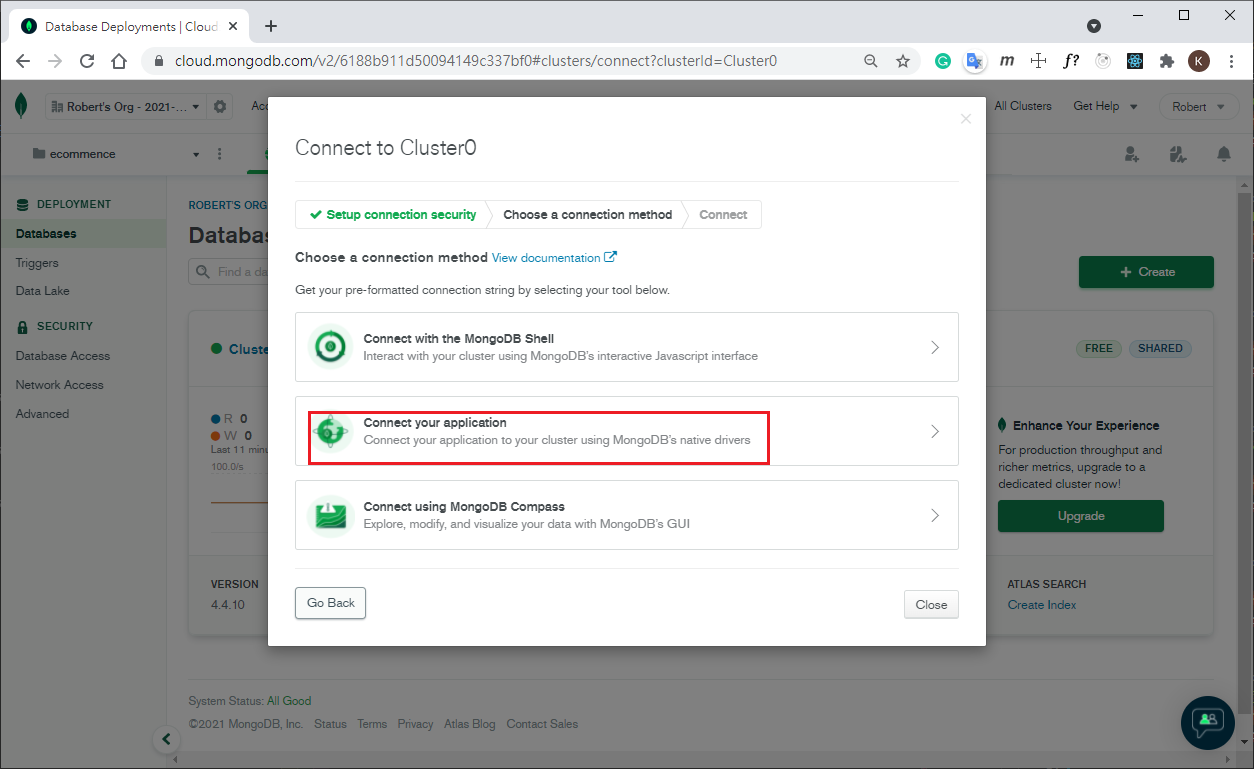
NOde.js 4.0 or later driver
使用時要填入正確的 password
myFirstDataBase 為 default DB name, 可改為其他名稱
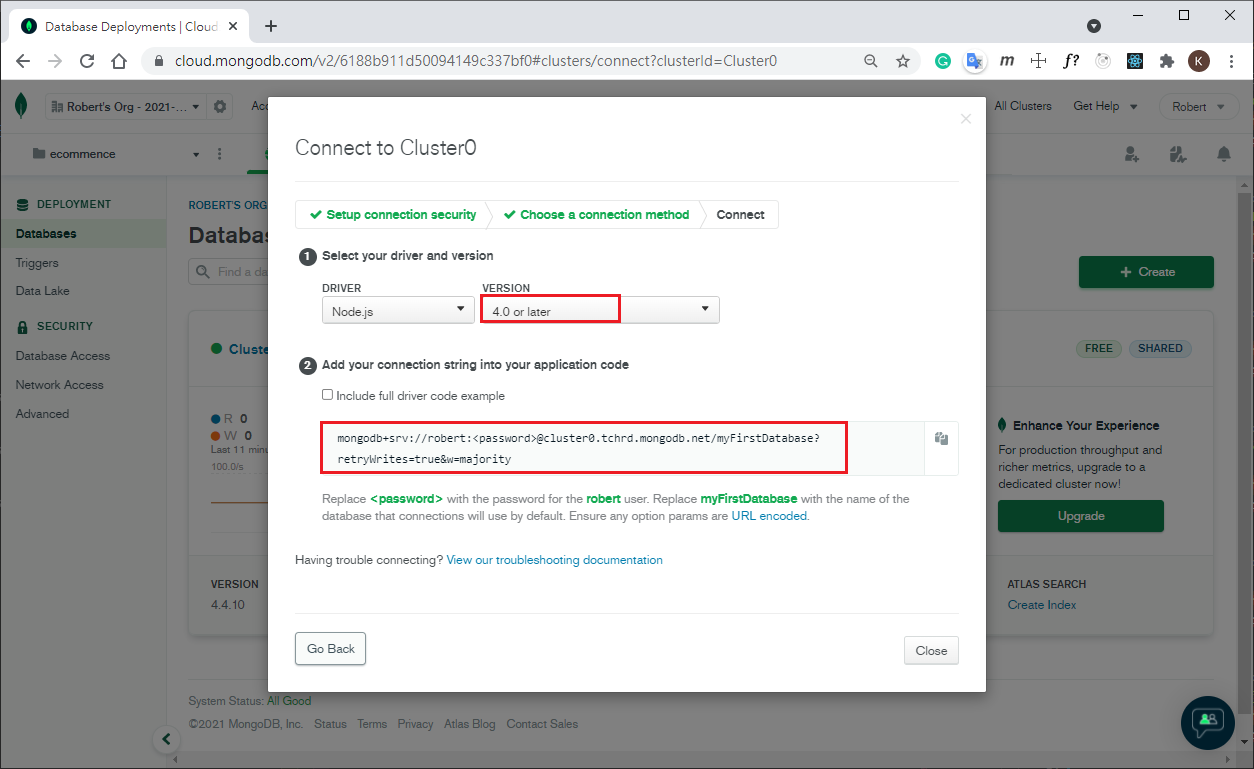
NOde.js 2.2.12 or later driver(某些applicat要使用這個版本才能使用)
使用時要填入正確的 password
myFirstDataBase 為 default DB name, 可改為其他名稱
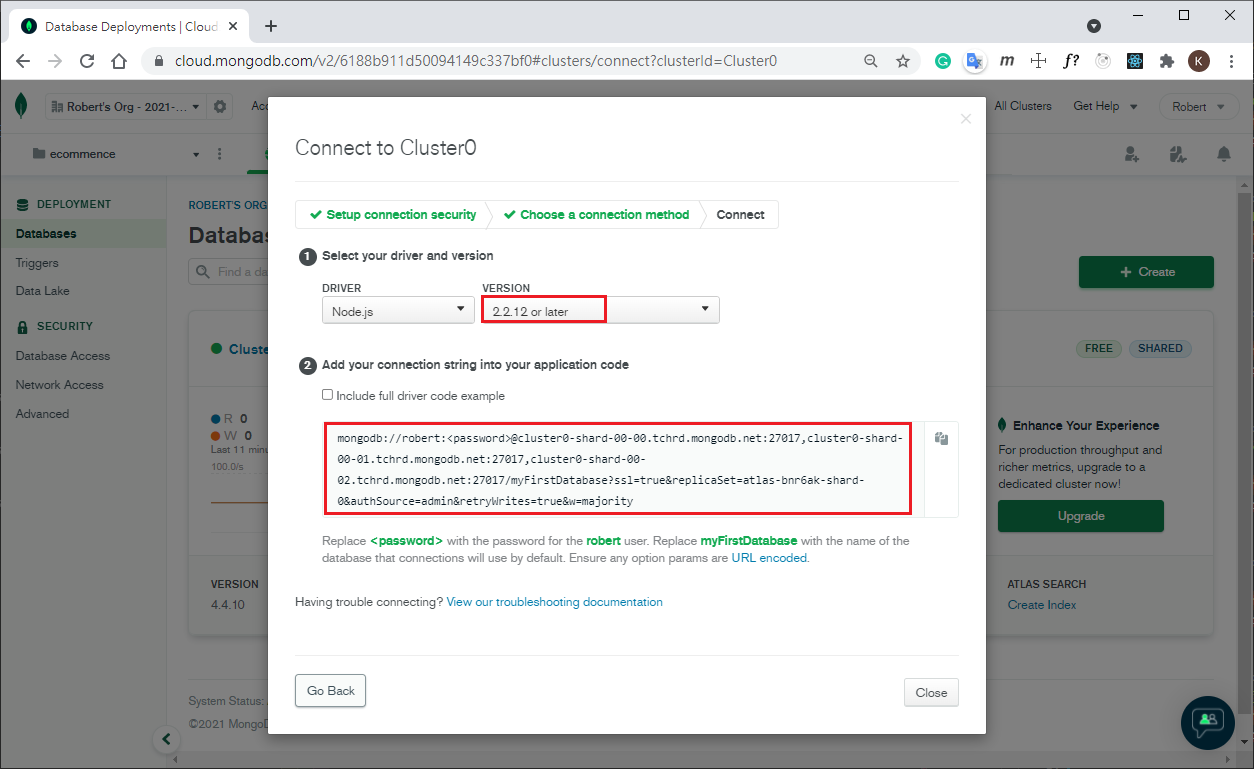
加入 database, Databases : add My Own data, database info+Create
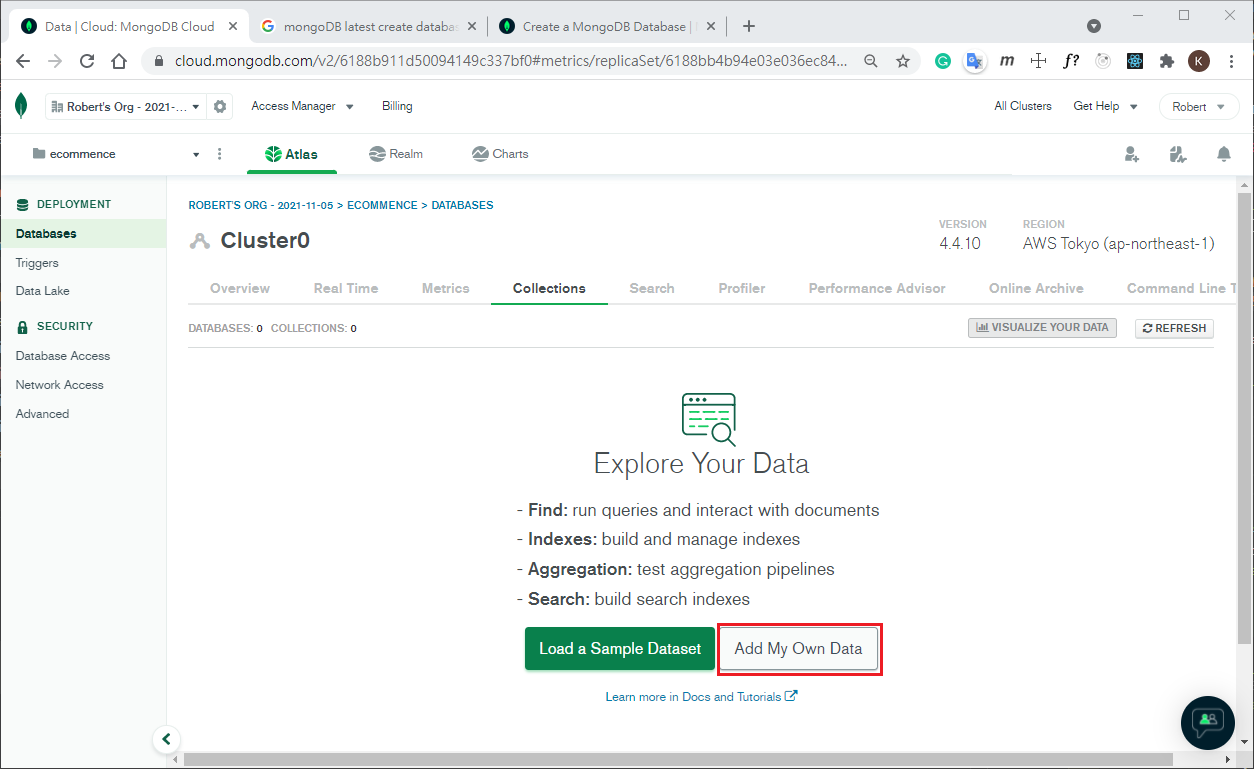
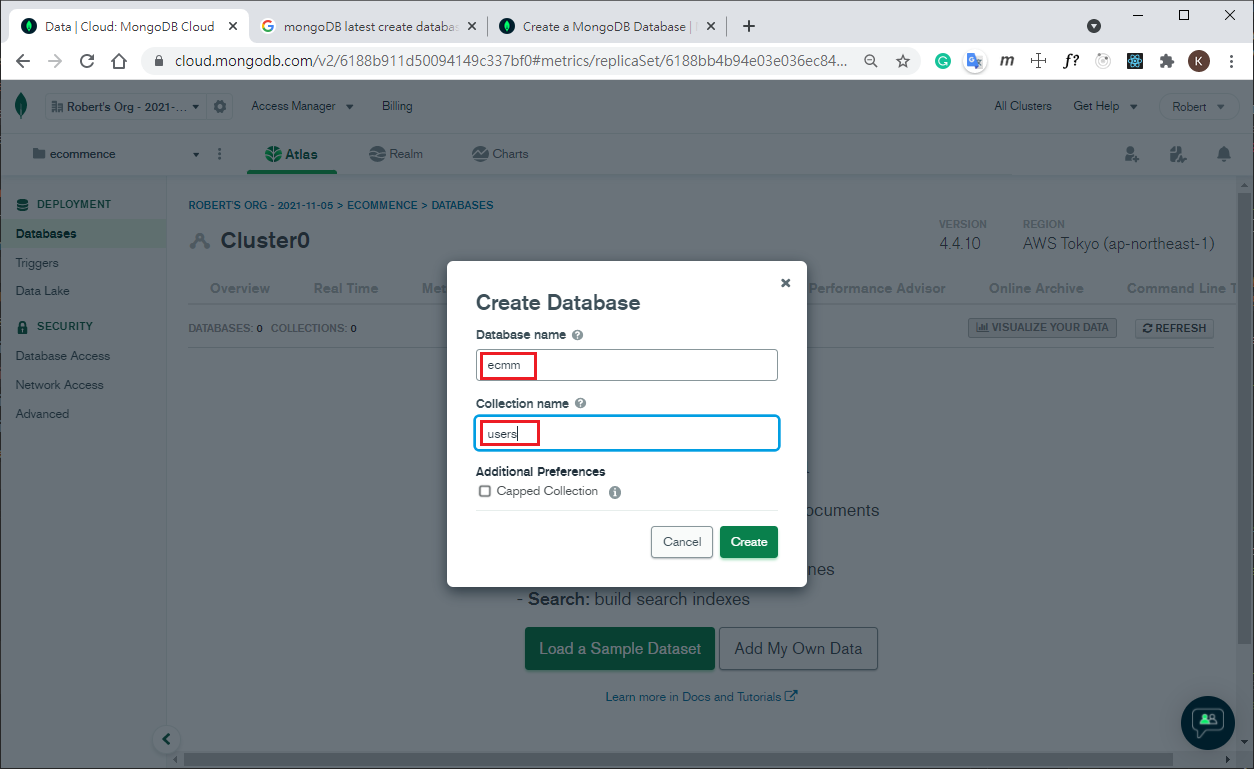
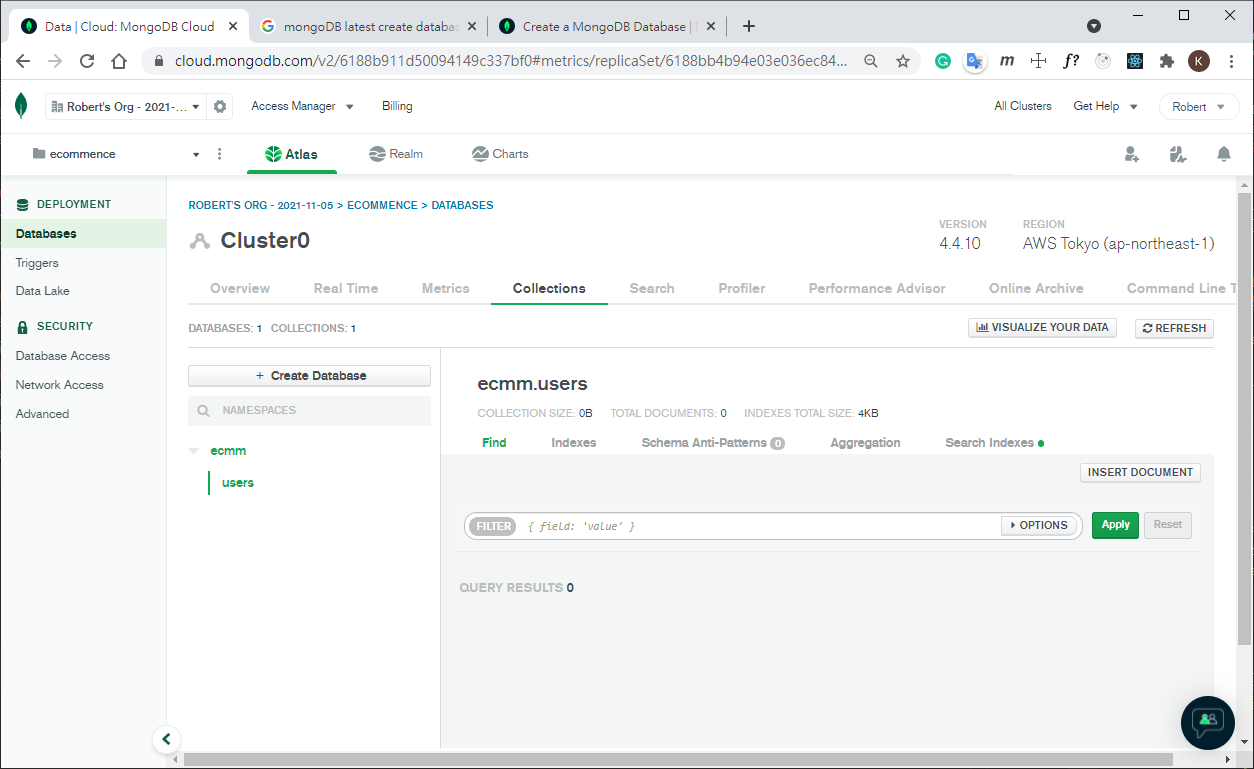
MongoDB
Schema
type
- String
- Number
- Array
- ObjectId
- Boolean
special
map to other
1
2
3
4
5category: {
type: ObjectId,
ref: "Category",
required: true,
},photo
1
2
3
4photo: {
data: Buffer,
contentType: String,
},simple string
1
salt: String,
default
- 0
- []
othert field
- trim : true
- required: true
- maxlength: 32
- unique: 32
- timestamps: true
$regex
1 | const query = {}; |
control
- Category.find().exec((err, data) => {}) : find all
- Product.find({ _id: { $ne: req.product }, category: req.product.category }): find by consition, $ne: not include
- Product.find(query, (err, products) => {}) : find direct run, not run exec
- findById(id).exec((err, product) => {} ) : find by id
- select(“-photo”) : remove field
- populate(“category”) : link to another table
- sort([[sortBy, order]]) : sort
- skip(skip) : set skip
- limit(limit) : set limit
- remove((err, deletedProduct) => {} ) : remove
- findOneAndUpdate() : find + update
1
2
3
4
5.findOneAndUpdate(
{ _id: req.profile._id },
{ $set: req.body },
{ new: true },
(err, user) => {})
mongoose : mongoDB object modeling tool to work in an asynchronous environment. Mongoose supports both promises and callbacks.
example code
1 | // connect mangoDB altas |
Deprecation Warnings
update()
1 | // Replace this: |
remove()
1 | // Replace this: |
count()
1 | // Replace this: |