Express
node.js create web server
1 | // index.js |
Express server
Example #1
1 | # install express |
1 | // index.js |
Example #2 - add template
1 | # template engine --> ejs |
1 | // index.js |
1 | <!-- ./views/todos.ejs --> |
1 | <!-- ./views/todo.ejs --> |
Example #3 - MVC
1 | // index.js |
1 | // ./controllers/todo.js |
1 | // ./modules/todo.js |
Example #4 - add mysql
1 | // index.js |
1 | // db.js |
1 | // ./controllers/todo.js |
1 | // ./modules/todo.js |
1 | <!-- ./views/todos.ejs --> |
1 | <!-- ./views/todo.ejs --> |
express middleware(中介軟體)
example - simple ( query string)
1 | // index.js |
1 | // ./controllers/todo.js |
1 | // ./modules/todo.js |
body parser
1 | # install body parser |
1 | // index.js |
1 | // ./controllers/todo.js |
1 | // ./modules/todo.js |
1 | <!-- ./views/todos.ejs --> |
1 | <!-- ./views/todo.ejs --> |
1 | <!-- ./views/addTodo.ejs --> |
express session
1 | # install |
1 | // index.js |
1 | // ./controllers/todo.js |
1 | // ./modules/todo.js |
1 | <!-- ./views/todos.ejs --> |
1 | <!-- ./views/todo.ejs --> |
1 | <!-- ./views/addTodo.ejs --> |
1 | <!-- ./views/login.ejs --> |
connect-flash
- connect-flash
- add global variable - use middleware
1 | # install |
1 | // index.js |
1 | // ./controllers/todo.js |
1 | // ./modules/todo.js |
1 | <!-- ./views/login.ejs --> |
簡易會員註冊系統
1 | # insatll bcrypt |
1 | // index.js |
1 | // ./controllers/user.js |
1 | // ./modules/user.js |
1 | <!-- ./views/user/index.ejs --> |
1 | <!-- ./views/user/register.ejs --> |
1 | <!-- ./views/user/login.ejs --> |
簡易留言板
1 | // index.js |
1 | // ./controllers/comment.js |
1 | // ./modules/comment.js |
1 | <!-- ./views/user/index.ejs --> |
1 | <!-- ./views/user/update.ejs --> |
ORM 與 Sequelize
ORM : Object Relational Mapping
Sequelize 基本操作
insatll sequelize
1 | npm install sequelize |
link DB and create table’s item
1 | Sequelize = require('sequelize'); |
get/modify/delete table item
1 | // create tabel item |
related db control
1 | // define another table field |
Sequelize CLI
install sequelize-cli
1 | # install |
init sequeliz
1 | # init sequeliz |
config/config.json
change db configuration
1 | { |
create model
1 | # npx sequelize-cli model:generate --name User --attributes firstName:string,lastName:string,email:string |
migrate (generate db table)
1 | # 產生 table sequelizemeta, 存執行 log |
為 model 加上關聯
./models/user.js
1 | ; |
./models/comment.js
1 | ; |
create table item
index.js
1 | const db = require('./models') |
改造留言板
data 操作
1 | // 在 ejs 可直接使用 |
1 | // migration - set field unique |
install and create DB(migrate)
1 | # install |
add user id for comment
./migrations/xxx-create-comment.js 加 user id
1 | ; |
set username unique
./migrations/xxx-create-comment.js set username unique
1 | username: { |
撤銷migrate then migrate
1 | # 撤銷 上一次 |
model 加上關聯
./model/user.js
1 | ; |
./model/comment.js
1 | ; |
index.js
1 | // index.js |
view’s template
template/head.ejs
1 | <meta charset="UTF-8"> |
template/navbar.ejs
1 | <nav class="navbar navbar-expand-lg navbar-light bg-light mb-3"> |
view
user/index.ejs
1 | <!-- ./views/user/index.ejs --> |
user/register.ejs
1 | <!-- ./views/user/register.ejs --> |
user/login.ejs
1 | <!-- ./views/user/login.ejs --> |
user/update.ejs
1 | <!-- ./views/user/update.ejs --> |
control
controllers/user.js
1 | // ./controllers/user.js |
controllers/comment.js
1 | // ./controllers/comment.js |
Ngainx and PM2
PM2
command
1 | # install |
Nginx
install
1 | # install nginx |
run web server
index.js
1 | const express = require('express') |
index2.js
1 | const express = require('express') |
1 | # start web server |
set for DNS
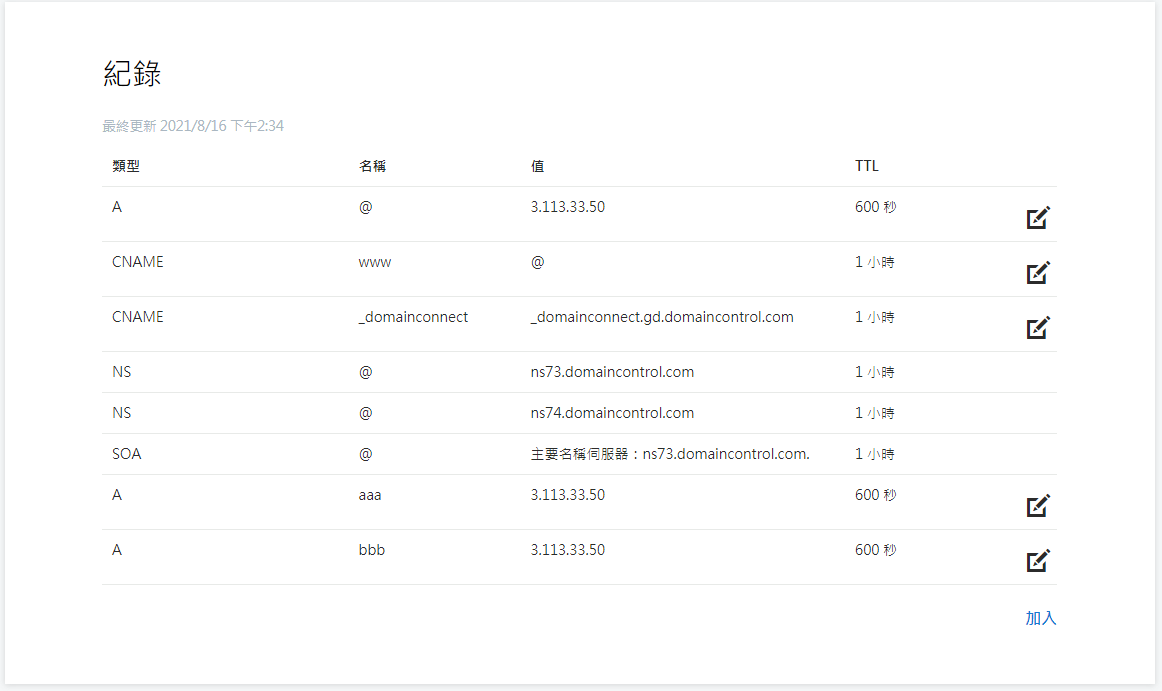
configure nginx to web server
1 | sudo vim /etc/nginx/sites-available/aaa.website |
aaa.website
1 | server { |
bbb.website
1 | server { |
add soft link
1 | sudo ln -s /etc/nginx/sites-available/aaa.website /etc/nginx/sites-enabled/ |
reload nginx
1 | sudo systemctl reload nginx |
Heroku
develop node.js - no db
install Heroku CLI
1 | heroku -v |
package.json add “script start” and “engines”
1 | "scripts": { |
set env port
app.js
1 | const port = process.env.PORT || 5001 |
run heroku local test
1 | heroku local web |
develop to heroku
add .gitignore
1 | node_modules/ |
git init and commit
1 | git init |
push to heroku
1 | # login |
develop node.js - include db
install Heroku CLI
1 | heroku -v |
package.json add “script start” and “engines”
1 | "scripts": { |
set env port
index.js
1 | const port = process.env.PORT || 3000 |
run heroku local test
1 | heroku local web |
develop to heroku
add .gitignore
1 | node_modules/ |
git init
1 | git add . |
push to heroku
1 | # login - if never login |
develop db for heroku
1 | # show logs for debug |
check models/index.js see use_env_variable for db parameter
1 | let sequelize; |
check heroku db env name
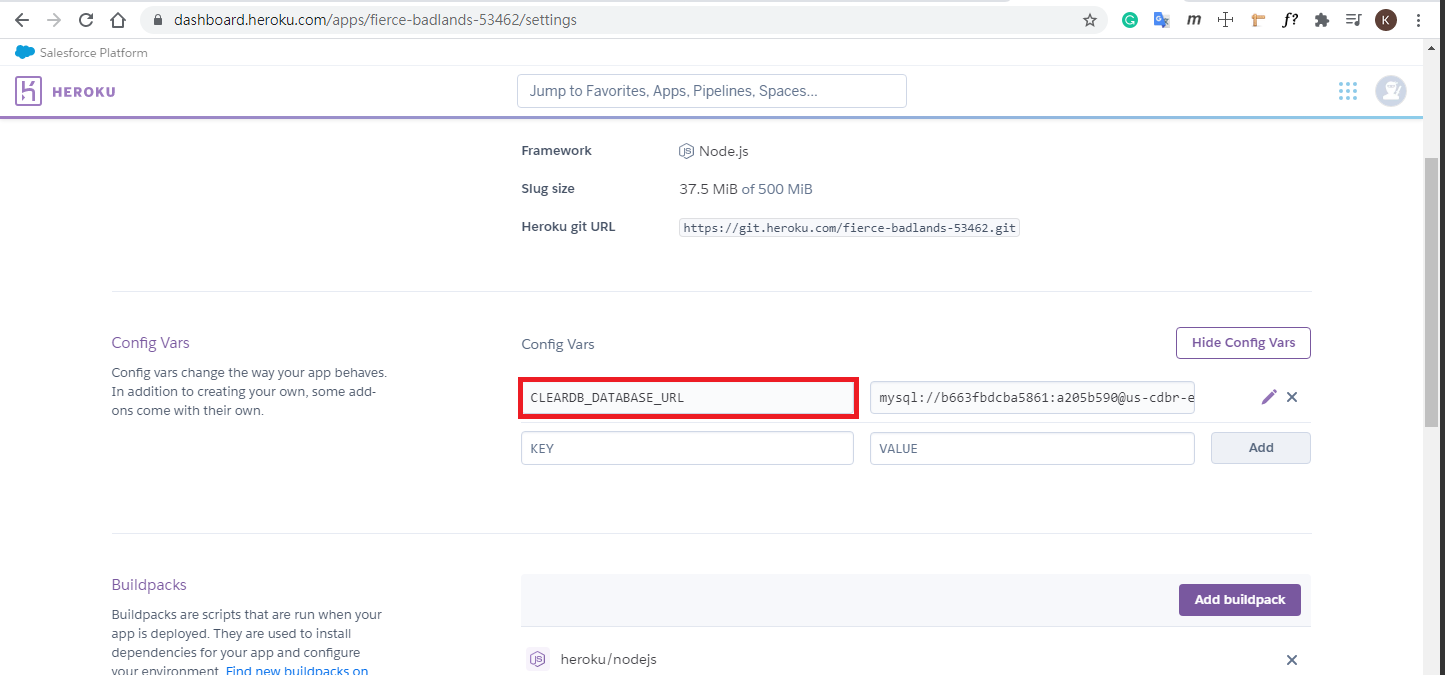
set config/config.json
1 | "production": { |
commit + push to heroku
1 | git add . |
add migrate script to package.json
1 | "scripts": { |
commit + push to heroku
1 | git add . |
heidisql link to heroku db
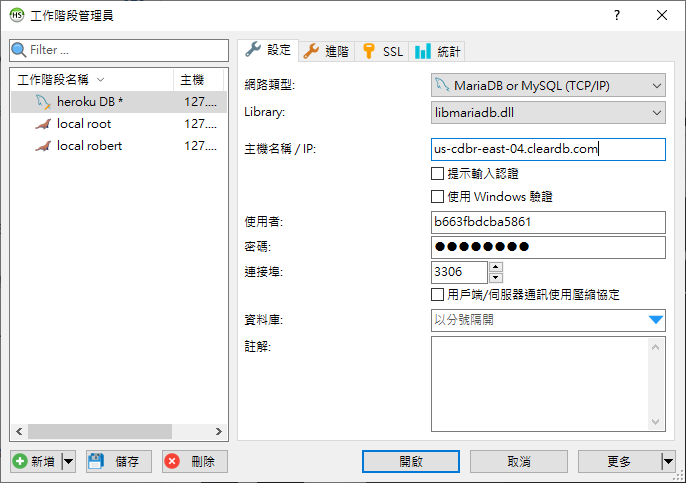
AWS 部署(node.js + express + nginx)
install nginx, node.js, npm, pm2
1 | # install nginx |
install mysql
1 | # Install MySQL |
其他 app 連至 MySQL
Inbound Rules 新增 HTTP TCP port:3306
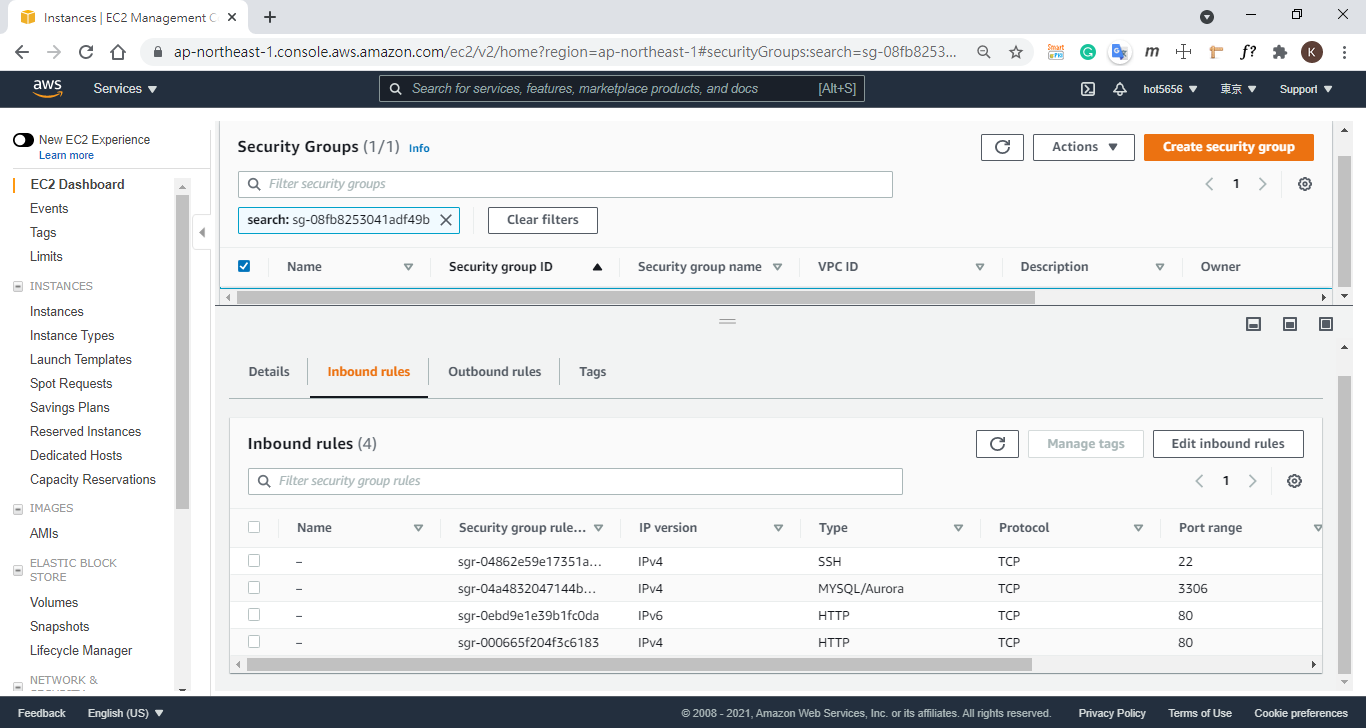
mask bind-address(允許連線主機)
1 | sudo vi /etc/mysql/mysql.conf.d/mysqld.cnf |
root localhost 改成 %
1 | sudo mysql -u root -p |
1 | # restart mysql |
heidisq port 3306 setting
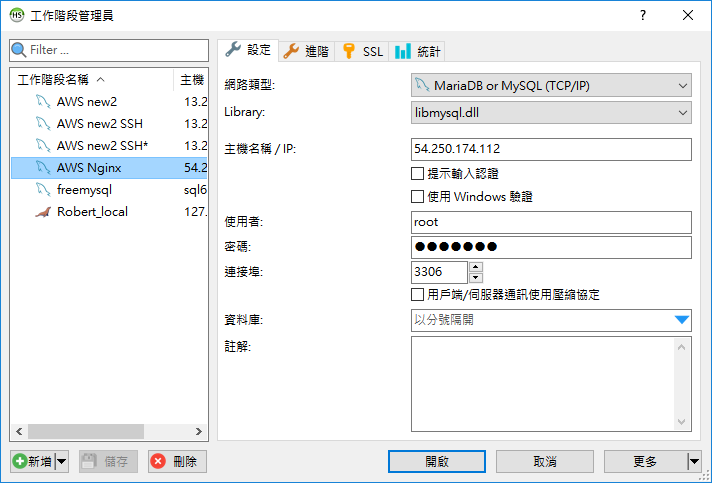
install and start app
1 | # ftp load code ex3-sequelize |
run nginx
configure nginx to web server
1 | sudo vim /etc/nginx/sites-available/aaa.website |
aaa.website
1 | server { |
bbb.website
1 | server { |
add soft link
1 | sudo ln -s /etc/nginx/sites-available/aaa.website /etc/nginx/sites-enabled/ |
reload nginx
1 | sudo systemctl reload nginx |
npm mysql
example #1
1 | # install mysql |
1 | // db.js |